JSON to Python (Reading In JSON Files)
- We use Python’s built-in JSON module with JSON files. Import the JSON module using the following import statement:
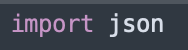
- Use one of the two methods from the JSON module to read in JSON data in Python.
The loads method parses a string of JSON code and turns it into a Python dictionary
jsonstring2dict = json.loads(‘jsonstring’)
The load method translates the data in a JSON file into a Python dictionary.
with open(‘jsonfilename.json’, ‘r’) as f:
json2dict = json.load(f)
EXAMPLE 1 (loads Method)
We have a JSON string we are attempting to read in and convert to a Python Dictionary.
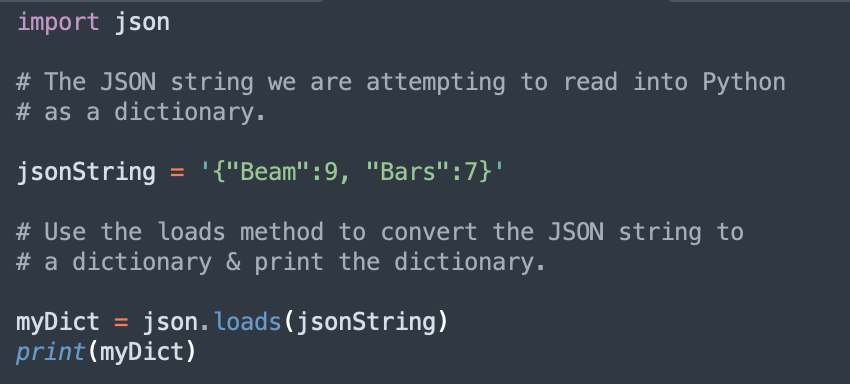
Output (a Python Dictionary):

EXAMPLE 2 (load Method)
Here, we have a JSON file we wish to convert into a Python dictionary.
Download sample.json
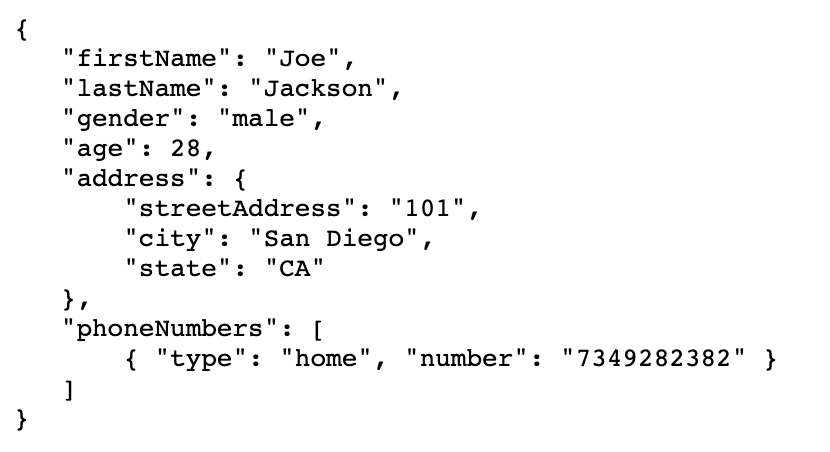
There are two steps to read in a JSON file:
- Open the JSON file
- Load the file using the load method
In Python, we can do both of these steps at once like this:
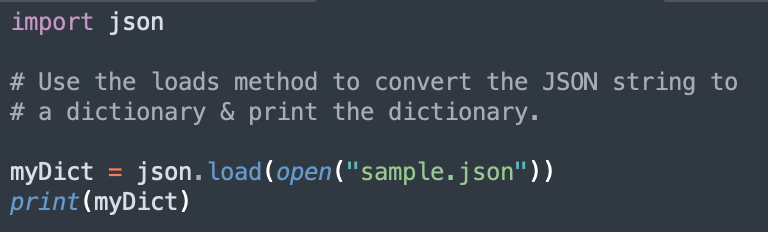
Output (Python Dictionary):
